When creating a webpart, there are a few steps that you’ll need to follow.
1. Create your project (duh).
2. Add 2 xml files. Filename.webpart and manifest.xml.
3. Add a text file and change the extension to ddf. I tend to call this file Solution.ddf.
4. Add the necessary references.
5. Strongly name your assembly.
6. Deploy
Ok, now, before we start following these steps, what do we need installed on our machines?
Assuming you already have a copy of Visual Studio and WSS, all you’ll need is the Microsoft Cabinet SDK. You can also download a copy of reflector. It’s a handy little tool. You don’t need it, but I’ll be using it in my demo’s. I currently use the one from Red-Gate.
Alright, once you have the Cabinet SDK and reflector, we’ll be ready to go.
First thing you’ll want to do is create your HelloWorld project. You’ll notice a few things in the image below. I’m using a Class Library, but if you look above, you’ll see that there are templates for the various Definitions, a Web Part template and an Empty one. You can get these templates on microsoft here. I’ll use them for future projects but in this particular project, I won’t be using them because I want to show you how to manually package and deploy a solution.
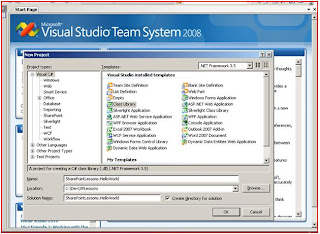
So if you look above, my solution is called “SharePointLessons.HelloWorld” and Create directory for solution is checked. Once you name it, hit ok and we’ll be ready to start.
When you’re solution is created, you’ll have one class file called Class1.cs. What we’re going to do is rename this file to Hello.cs and place it in a new folder called HelloWorldWP. After you do this, add 2 xml files to the HelloWorldWP folder. HelloWorld.webpart and manifest.xml. While we’re at it, add a text file to your project (outside of the HelloWorldWP folder) and call it Solution.ddf. When you’re done, you’re project should look like this.
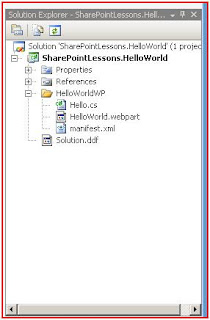
Next, we’ll want to add our references. You’ll see an item in the image above labeled “References”. Right-click on that and select “Add Reference”. A window will open and you’ll want to select “System.Web” from the .NET tab and click OK.
Now, we’re ready to start filling in the files. Let’s start by opening the Hello.cs and adding the following lines:
using System.Web.UI.WebControls;
using System.Web.UI.WebControls.WebParts;
Next, our class will inherit from the WebPart class (System.Web.UI.WebControls.WebParts.WebPart). Once we add the inheritance, we’re going to add a Label control (this comes from System.Web.UI.WebControls).
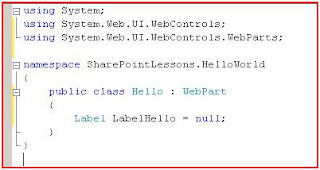
Once you’re class looks like the image above, you’ll want to override the CreateChildControls method. To do this, underneath your label control, type the word override and add a space after it. Intellisense will pop up and you’ll want to select CreateChildControls and hit the tab key.
You’re class should look like this.
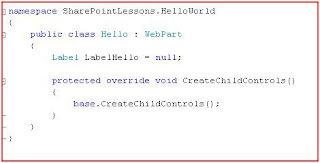
Now you’re going to want to start setting up your label. We’re going to instantiate the label inside the CreateChildControls method and set the text, font size, and font weight. Your CreateChildControls method should look like the following.
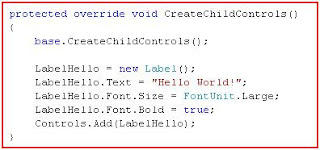
Everything in this method about the label properties should be self explanatory. At the end of the method, there’s a “Controls.Add(LabelHello)”. This line will display your label in the webpart. You can also override the RenderContents method to play with the position of your controls but that’s a future lesson.
Ok, so far so good. The next 3 files can be tricky. We’re going to start with the HelloWorld.webpart file. Open this file and you’ll want to add a few elements. I’m going to get straight to the point and show you the final result (b/c my fingers are getting tired).
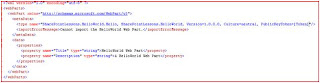
Be careful with this file. It’s case sensitive. Basically, every webpart will look like this. In “”, you’ll see a type node w/ a long name attribute. This name is the Namespace.Class, Namespace, Version, Culture, PublicKeyToken. Where you see “[Token]”, you’ll have to replace w/ the actual key. We’ll do this later.
The importErrorMessage will display “Cannot import the HelloWorld Web Part” if the webpart cannot be added to your page. In this example, if you see this message, it will most likely be due to a typo in one of the xml files.
Finally, the 2 properties will be used to give your webpart a Name and Description for the user when they’re adding it to a page.
Now, we’ll move on to the manifest.xml file. There are a few important things in this file. First is the Assembly node. We have 2 attributes here.
1. DeploymentTarget: can contain 1 of 2 values. GlobalAssemblyCache or WebApplication
2. Location: this is the assembly name. In this case, if I build my project, I’ll have a dll called “SharePointLessons.HelloWorld.dll”.
Next, within the Assembly node are your SafeControl entries. This contains the same info as the type node’s name attribute in the .webpart file in a slightly different format.
Finally, the DwpFiles. In the DwpFile Node, you’ll have 2 options. You can either use the Filename attribute or the Location attribute but this depends on the type of file that you’re using. Since we’re using a .webpart file, you’ll need to use Location. If we had a .dwp file (this is the format used in v2), you’ll need to use the Filename attribute. So our Location attribute is going to have the name of our .webpart file.
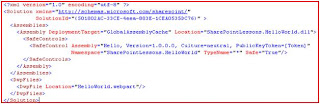
Hang in there. We’re almost done. All we need to do now is set up our ddf file and sign our assembly. (Oh, and we’ll have to get our key from our assembly and replace the 2 [Token] placeholders.)
Alright, here we go. The ddf file is going to be used to create our wsp file. The wsp file is essentially a cab file used package all the work we just did and it’ll be passed to SharePoint. When deployed, it’ll put our files where they need to go.
So, if your webparts are always structured the way we’ve structured it here, this file will always be set up this way. What you need to worry about here is the CabinetNameTemplate. This will be the name of your solution (WSP). You can call it whatever you want. DiskDirectory1 will be used to create a folder that will contain your fully construction solution file. The last 3 lines are the files that we’ve spent most of our time setting up. You have the manifest and the .webpart files located in the HelloWorldWP folder and then the location of the assembly when you build this project.
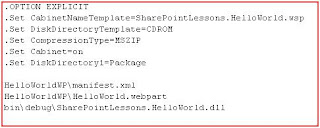
Now we’ll sign our assembly. Right-click on our project and select Properties.
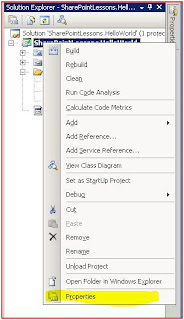
Click on Signing in the left nav. Check the Sign the assembly box. Select New from the drop down and name your key file. I named mine KeyFile.snk (very creative right). I also unchecked the Protect my key file with a password. Alright people, you should notice your key file in your solution explorer window and once you build your project (Build Now) our assembly will be signed. Now let’s get the PublicKeyToken. Open reflector (one of the apps that I mentioned in the beginning) and click on the Open menu item in the File menu. When the Open Assembly window opens, find your DLL and click on open.
Your assembly will be added to the tree and when you select it, you’ll see your assembly info. I’ve highlighted it in the image.
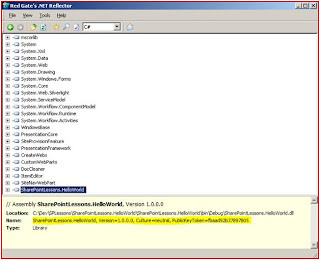
You’ll see your token at the end of the highlight. Go back and replace the token placeholders in the .webpart and the manifest files. Save everything and now we’re ready to create our wsp, add our solution to the solution gallery and deploy it to our site(s).
Open the command prompt and create the WSP:
1. Go to the path where your solution.ddf is located. In my case, My prompt shows:
“C:\dev\SPLessons\SharePointLessons.HelloWorld\SharePointLessons.HelloWorld>”
2. Type: makecab /f Solution.ddf
Now if you look at the directory where your solution is stored, you’ll notice a Package folder. In this folder, you’ll find your wsp.
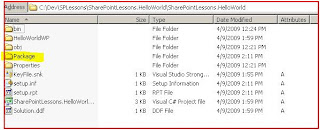
Now let’s Add the solution to the solution store.
1. Open another command prompt and go to the following path:
“C:\Program Files\Common Files\Microsoft Shared\web server extensions\12\BIN”
2. Type stsadm –o AddSolution –filename [path to your Package\SharePointLessons.HelloWorld.wsp]

If you open Central Administration, click the Operations tab, and select “Solution management” in the Global Configuration section, you’ll see “sharepointlessons.helloworld.wsp”.
Finally, let’s deploy. If you went to the Central Admin location, you can click on your wsp and you’ll have options to Deploy to specific web applications. Very straight forward stuff. Just make sure you select the right web application. You can also do this using the command line. Instead of an addSolution like we did above, we’ll want to do a deploy solution. So follow the same steps as above just change the stsadm part to show:“stsadm -o deploysolution -name SharePointLessons.HelloWorld.wsp -url http://yoursite -immediate -allowGacDeployment ”
Since we’re deploying to the gac, we need the –allowGacDeployment.
We’re done. Go to the site that you deployed your webpart to, click on the Site Actions menu and select Edit Page. You’ll see a button that says “Add a Web Part” at the top of each webpart zone. Click on one. The Add Web Parts window will open and you’ll need to go to the All Web Parts section. There will be a Miscellaneous section and you should find your webpart in there. It will say HelloWorld Web Part in the title and “A HelloWorld Web Part” in the description (if you copied what I put in my .webpart file). Click the Add button and your webpart will be dropped on the page.
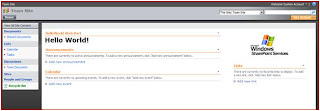
We’re done people. This was a long post since it involved the full details on creating a webpart from scratch. Future posts will skip the full process. Now, if you download the SharePoint Extensions by Microsoft, they have the ability to create and deploy the wsp. I showed you the long way so that you know what is involved in the creation of a webpart.
Ok, I hope you enjoyed this lengthy post. Feel free to post comments, suggestions, whatever. (Just don’t bad mouth me, my fingers hurt after typing this for you.) Also keep in mind that this blog is intended for Devs and End Users. Sorry admins, I’m not strong enough on that side of SharePoint.Labels: Developer